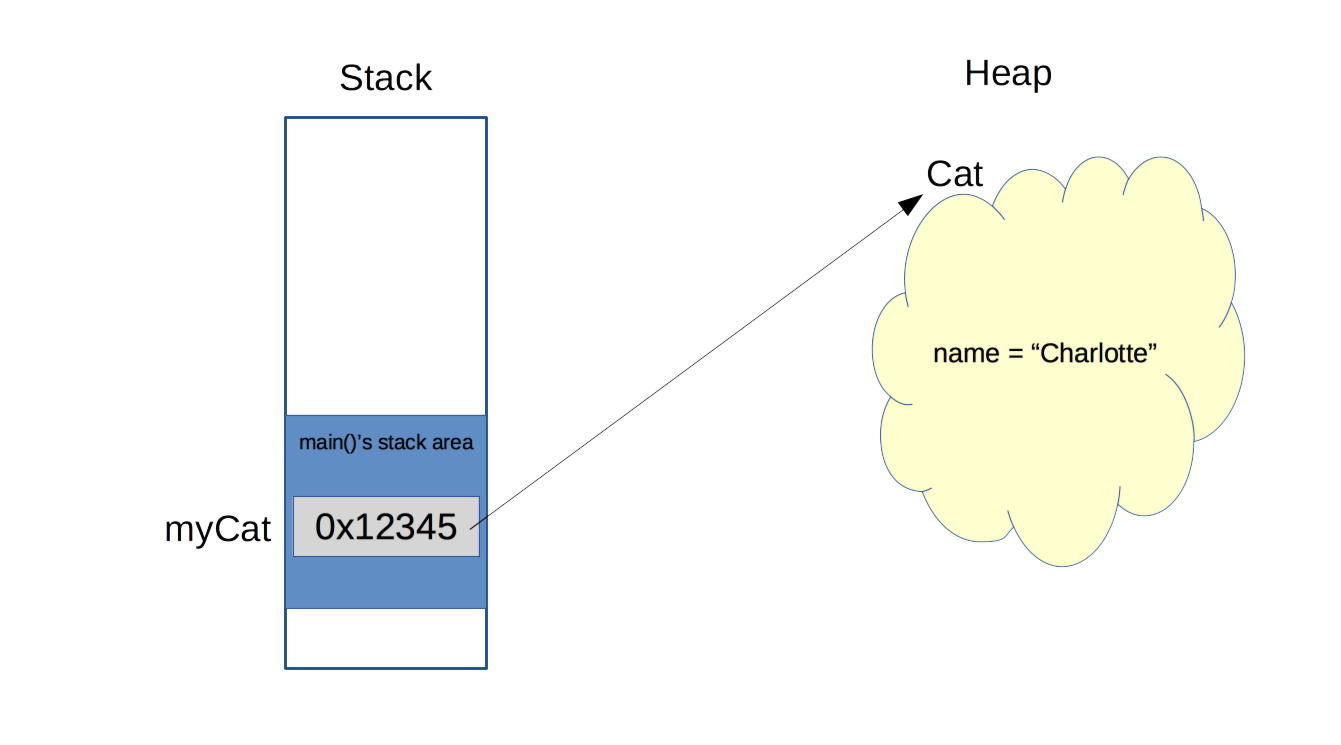
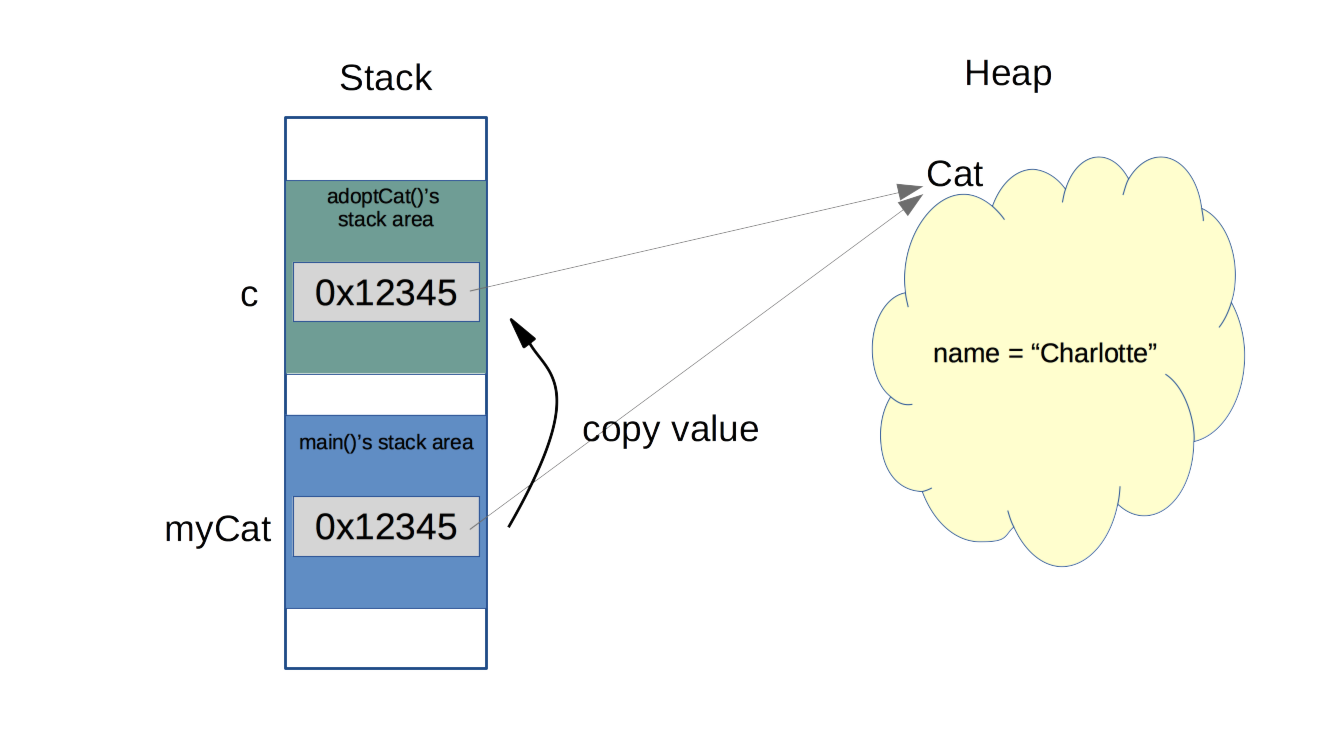

.element height="40%" width="40%"
--- ## Delegating 什么叫委托?举个不太合适的例子: ```java public class Car { public Window[] windows = new Window[4]; } public class Jetta extends Car{ } Car myCar = new Jetta(); //手摇式玻璃 myCar.windows[0].open(); ``` --- ## Delegating ```java public class Car { public Window[] windows = new Window[4]; } public class Tesla extends Car{ public void openWindow(int i){ this.windows[i].open(); //delegate } } Car hisCar = new Tesla(); //自动式玻璃 hisCar.openWindow(1); ```
.element: class="fragment"
.element: class="fragment"